C#控件DataGridView之六(常用功能)
常用基础功能
表头居中显示
1 | dataGridView1.ColumnHeadersDefaultCellStyle.Alignment = DataGridViewContentAlignment.MiddleCenter; |
通过修改表格控件.ColumnHeadersDefaultCellStyle.Alignment
的值,我们可以将表头靠左、靠右、居中。
数据居中显示
1 | foreach (DataGridViewColumn column in dataGridView1.Columns) |
遍历 DataGridView 的每一列,并将列的默认单元格样式的对齐方式设置为居中对齐,即
DataGridViewContentAlignment.MiddleCenter
,这样就可以实现居中显示的效果。
一次选中一整行
1 | dataGridView1.SelectionMode = DataGridViewSelectionMode.FullRowSelect; |
通过设置其 SelectionMode 属性来完成。
每一次只允许选中一行
1 | dataGridView1.MultiSelect = false; |
当将 MultiSelect
属性设置为 false 时,就只能每次选中一行。
获取选中行的数据
1 | if (dataGridView1.SelectedRows.Count > 0) |
首先检查是否有选中的行,如果有,则获取选中的第一行,并遍历这一行的每个单元格,获取单元格的值并进行处理(此处是将值打印到控制台)。
监听选中行发生改变的事件
1 | private void dataGridView1_SelectionChanged(object sender, EventArgs e) |
要在选中行发生改变时得到通知,可以使用DataGridView的SelectionChanged
事件。
在方法内部,可以通过dataGridView1.SelectedRows获取选中的行,并进行相应的处理。
设置行高和列宽
设置行高:
1 | dataGridView1.RowsDefaultCellStyle.Height = 30; // 将默认行高设置为 30 像素 |
设置列宽:
1 | dataGridView1.Columns["ColumnName"].Width = 100; // 将指定列名为"ColumnName"的列宽设置为 100 像素 |
通过索引设置列宽:
1 | dataGridView1.Columns[0].Width = 80; // 将第一列的列宽设置为 80 像素 |
通过条件筛选显示部分内容
可以通过以下步骤对 DataGridView 的内容进行条件筛选显示:
- 获取要筛选的数据来源,例如 DataTable 。
- 根据设定的条件对数据进行筛选。
- 将筛选后的结果重新绑定到 DataGridView 。
1 | DataTable data = (DataTable)dataGridView1.DataSource; // 获取数据源 |
标题/列内容完全居中及选中行突出显示
一、列标题居中
1.首先点击属性窗口的ColumnHeadersDefaultCellStyle属性进入属性设置子界面,并设置子界面属性Alignment的值为MiddleCenter(如下图)
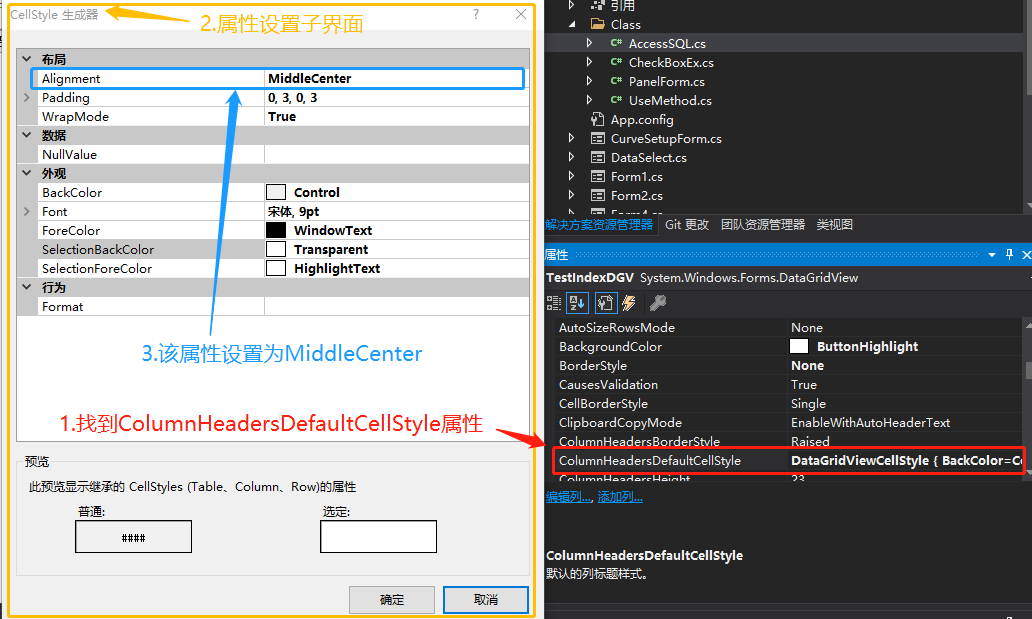
2.如果此时列标题未完全居中,那么找到列集合设置属性(Columns)进入子界面,将所有列头的SortMode属性设置为NotSortable(如下图)
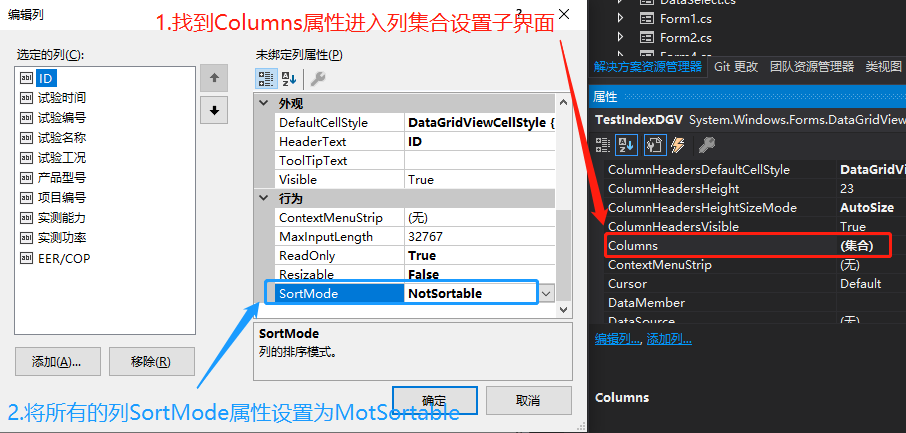
以上就是设置列标题居中的方法了
二、列值居中
只需在列集合设置属性(Columns)进入子界面,在子界面找到DefaultCellStyle属性,进入DefaultCellStyle属性设置子界面,将Alignment属性数值为MiddleCenter即可
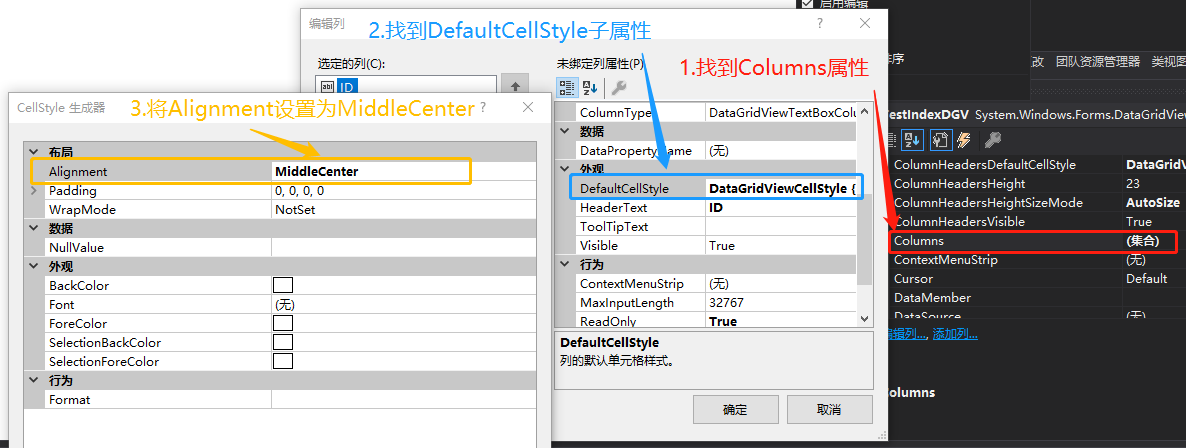
三、选中行突出显示
1.将SeltctionMode属性设置为FullRowSelect
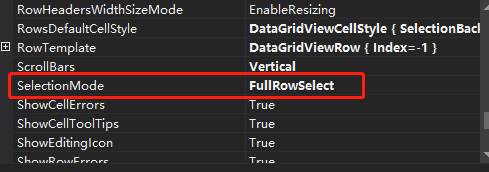
2.进入ColumnHeadersDefaultCellStyle子界面,将SelectionForeColor属性设置为Transparent(这里是为了不然选择时突出列标题)
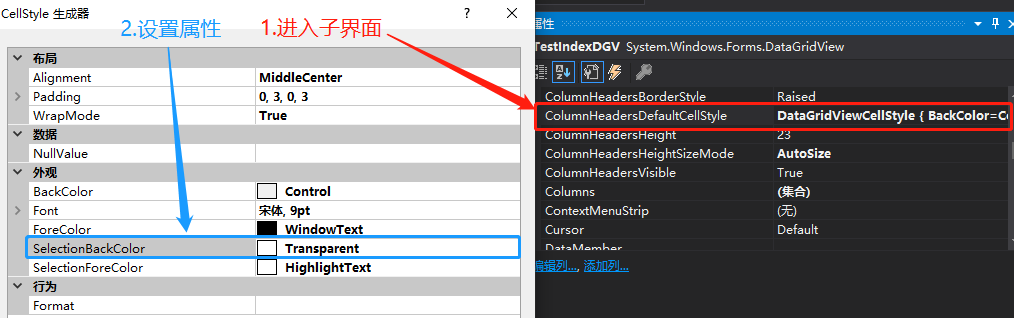
3.再进入RowsDefaultCellStyle子界面,将SelectionBackColor属性设置为Gray(这里是设置行选中时的颜色)
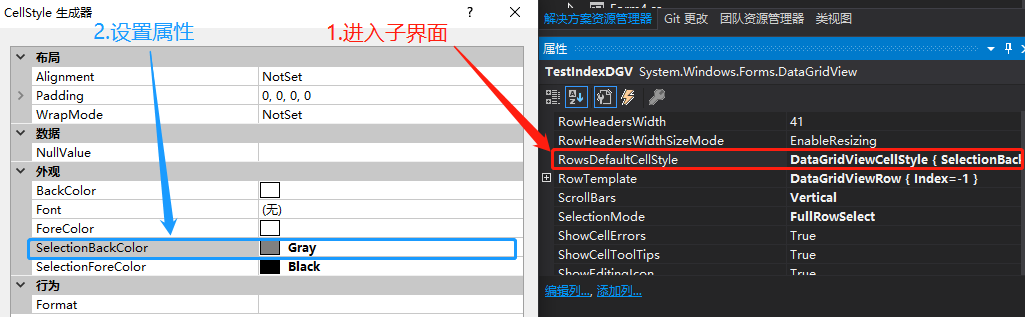
4.如果需要关闭多行选择的话将MultiSelect属性设置为False
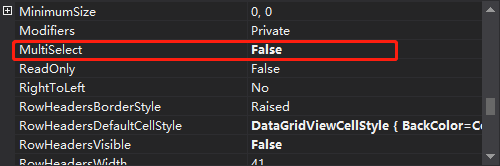
四.标题样式
如果未将EnableHeadersVisualStyles标志设置为False,则对标题样式所做的更改将不会生效,
dataGridView1.EnableHeadersVisualStyles = false;
dataGridView1.ColumnHeadersDefaultCellStyle.BackColor = Color.Blue;
相关链接(侵删)
欢迎到公众号来唠嗑:
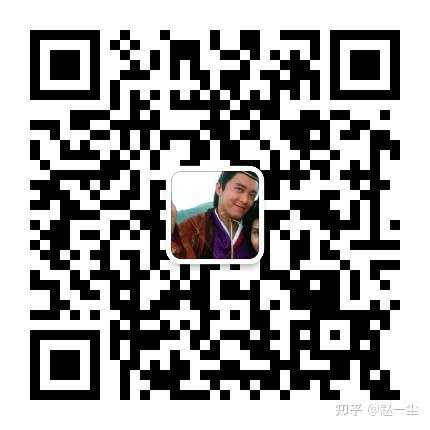